Published
- 2 min read
How to publish on Github Pages with create-react-app and react-router
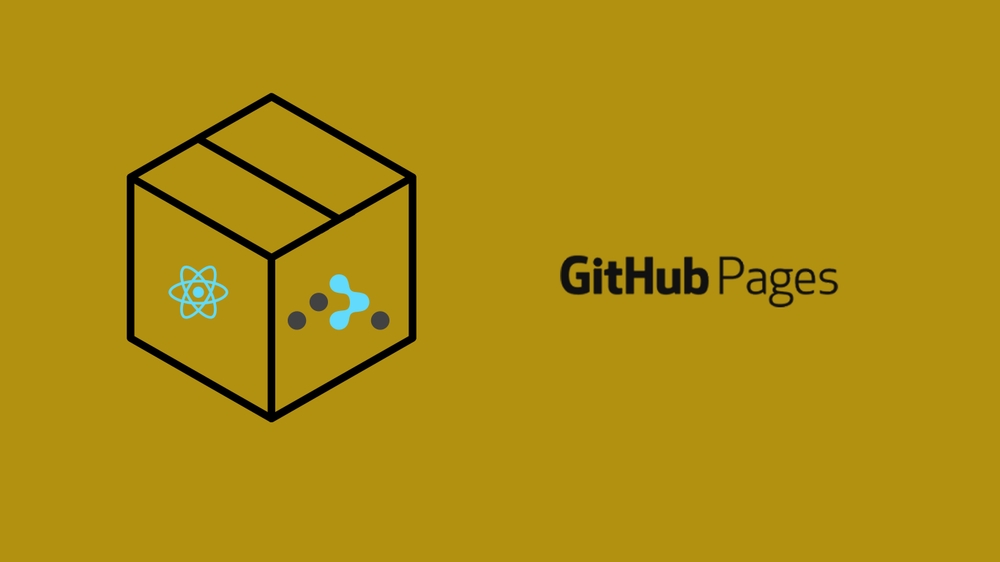
Now I will be assuming a couple of things:
- You have a GitHub account and you created a repository for your app.
- You created a react app either with
npx create-react-app <appName>
ornpx create-react-app <appName> --scripts-version=react-scripts-ts
- Then let’s get started.
Add gh-pages
Run npm i -D gh-pages\
Configure your package.json
First, you need to tell the react app where it will be hosted. You need to add to your package.json
the attribute ‘homepage’:
{ ...
"homepage": "https://<username>.github.io/<RepositoryName>/"
}
Additionally, you need to adjust the scripts attribute.
"scripts": {
...
"predeploy": "npm run build",
"deploy": "gh-pages -d build"
},
Now you can run npm deploy
and it will automatically create a branch, build the app and deploy it into your repository. It will even configure your github repository that the branch gh-pages will be now hosted. So you can simply navigate to https://<username>.github.io/<RepositoryName>/
and see the result. # Configure React-Router Before we can publish we probably have to change a couple of things in the configuration of the react-router.
- We need to set the basename. The basename ensures that all links of the Router are relative to this basename. To configure the basename you can use the variable
process.env.PUBLIC_URL
this ensures that you only need to configure the url via the package.json. - We need to ensure that we are using a
HashRouter
instead of the typicalBrowserRouter
. The difference is that the hashRouter adds a ’#’ into the url and upon a refresh of the page you will not see a 404 Github Error
Your index.js
should look something like this:
import * as React from 'react';
import * as ReactDOM from 'react-dom';
import { Router } from 'react-router';
import createHashHistory from 'history/createHashHistory';
const hashHistory = createHashHistory({ basename: process.env.PUBLIC_URL });
ReactDOM.render(
<Router history={hashHistory}>
<App />
</Router>,
document.getElementById('root') as HTMLElement
);
Deploy
Now can simply run `npm deploy` and your site should be published on https://<username>.github.io/<RepositoryName>/
Note: Since the build is published on a separate branch, your main repository may contain different src than what is published. So you still need to ensure that you commit your changes to your repository.