Published
- 2 min read
CodeWars: Weekly Newsletter
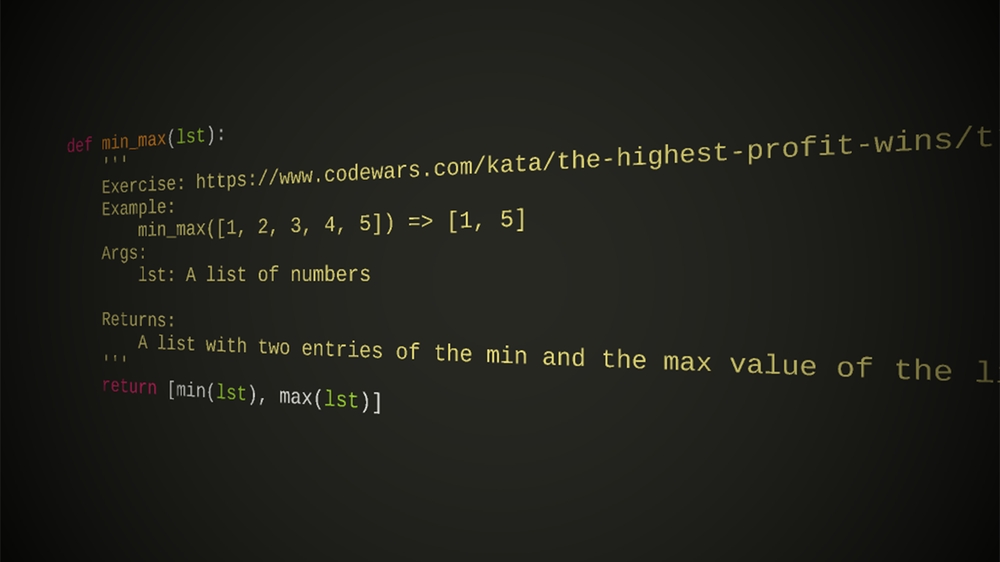
8kyu - positiveSum
The first question is to sum up all the positive integers in an array. Basically two things need to be done:
- Filter out Values that should not be added to the sum
- Sum up every item in the array
Basically you can write the solution like this:
export function positiveSum(arr: number[]): number {
return arr.filter((val) => val > 0).reduce((total, current) => total + current, 0)
}
It is important to note that the initial value of 0 must be set, as if you pass in an empty array it would return NaN
7kyu - next Prime
Basically you need to check every number if it isPrime
.
Then you need to select an algorithm that can check if it is a prime number.
In this case I just took the code from the npm package is-number-prime. Which is a brute force way of figuring out if the number is prime or not. (There is probably something more mathematically elegant out there)
function isNumberPrime(n) {
if (n === 2 || n === 3) {
return true
}
if (n < 2 || n % 1 || n % 2 === 0 || n % 3 === 0) {
return false
}
let foo = Math.floor(Math.sqrt(n))
for (let i = 5; i <= foo; i += 6) {
if (n % i === 0 || n % (i + 2) == 0) {
return false
}
}
return true
}
function nextPrime(n) {
while (true) {
n++
if (isNumberPrime(n)) {
return n
}
}
}