Published
- 2 min read
CodeWars: Weekly Newsletter 02
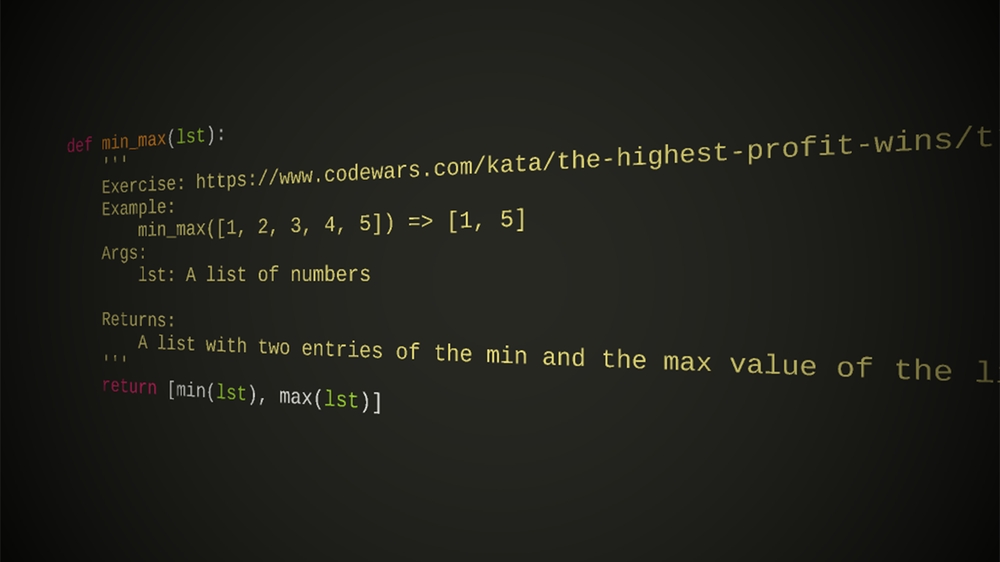
8kyu - Logic Drills: Traffic light
You need a function to handle each change from green, to yellow, to red, and then to green again.
Solution 1: Using Arrays
You could assume that you have an array of lights and the function will simply loop through the array. If it is the last item it should return the first item.
function updateLight(current) {
const lights = ['green', 'yellow', 'red']
const nextIndex = (lights.indexOf(current) + 1) % lights.length
return lights[nextIndex]
}
Solution 2: Using a Map
You can also look at the question as that for a specific light, a different light will be returned. i.e. it is a map. This is especially a valid solution where the array of lights will never change. Thus the solution is to use an object to save the next states.
function updateLight2(current) {
return {
green: 'red',
yellow: 'green',
red: 'yellow'
}[current]
}
7kyu - Sum of numerous arguments
Solution
The solution to this problem is to make aware of that you can pass in an arbitrary amount of arguments into a js function.
In ES5 you would be using the arguments
object and iterate over the array. in ES6 however we can use the cleaner spread-syntax:
function findSum(...args) {
if (args.find((val) => val < 0)) {
return -1
} else {
return [...arguments].reduce((total, current) => total + current)
}
}