Published
- 2 min read
Exercise of the Day: Determine if String Halves Are Alike
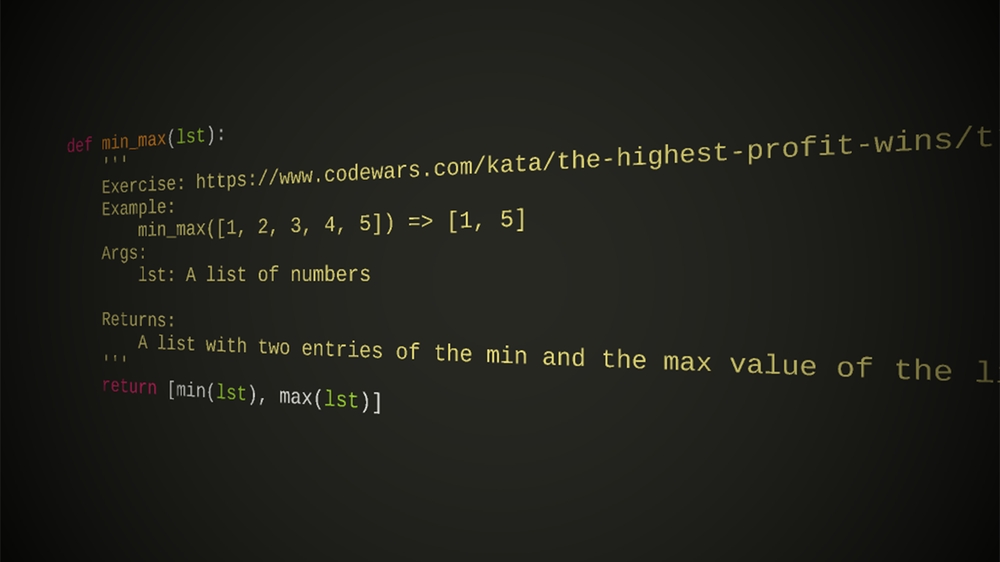
Problem:
You are given a string s of even length. Split this string into two halves of equal lengths. Two strings are alike if they have the same number of vowels
Solution
The question already spells out the different steps:
- Split the string in half
- Count the vowels in both strings
- Compare count of both strings
Split String into Half
Instead of creating a function that only can split a string in two halves, we should consider creating a generic splitStringAtPosition
and a second function splitStringInHalf
.
export function splitStringAtPosition(s: string, pos: number): string[] {
return [s.substr(0, pos), s.substr(pos)]
}
export function splitStringInHalf(s: string): string[] {
return splitStringAtPosition(s, s.length / 2)
}
Counting Vowels
My original solution filtered the array and then asked for the length.
export function countVowels(s: string): number {
const vowels = ['a', 'e', 'i', 'o', 'u', 'A', 'E', 'I', 'O', 'U']
return [...s].filter((val) => vowels.includes(val)).length
}
However you probably should use reduce
instead to make it more readable.
export function countVowels2(s: string): number {
const vowels = ['a', 'e', 'i', 'o', 'u', 'A', 'E', 'I', 'O', 'U']
return [...s].reduce<number>((acc, val) => {
return acc + Number(vowels.includes(val))
}, 0)
}
Final Result
Now we can put everything together and end up with this very readable function
export function halvesAreAlike(s: string): boolean {
const [a, b] = splitStringInHalf(s)
return countVowels(a) === countVowels(b)
}