Published
- 2 min read
Embracing TypeScript with Node.js
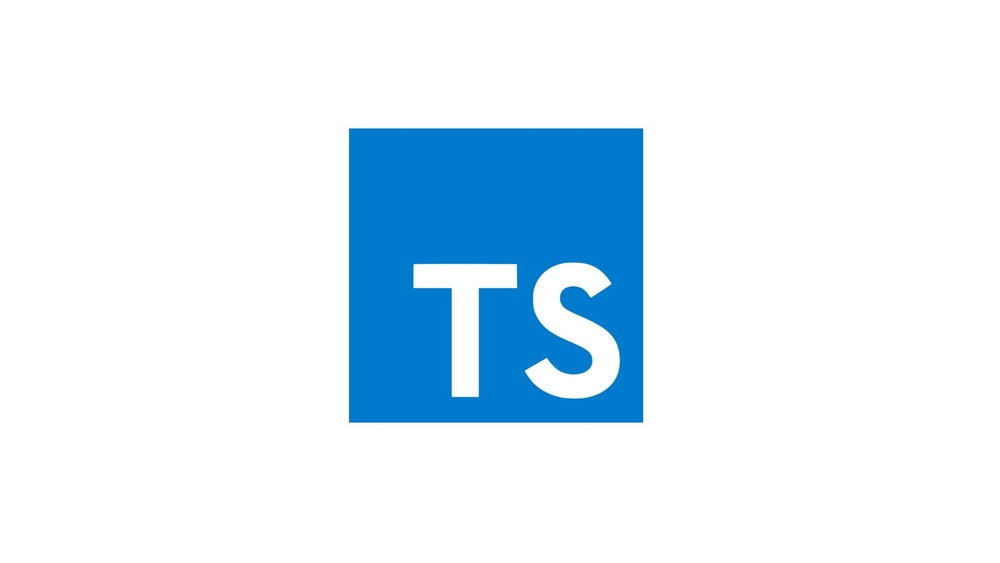
Recently, Node.js 23.6.0 was released, introducing a significant change: the flag --experimental-strip-types
is now enabled by default. This development allows Node.js to run TypeScript files without additional tools.
This is a very big deal, since now NodeJS can run TypeScript files without any additional tools.
This sounds too good to be true right? It’s important to note that not all TypeScript constructs can be removed through type stripping.
That means constructs like the following are not supported: -
enum
declarations -namespace
s andmodule
s with runtime code - parameter properties in classes - Non-ECMAScriptimport =
andexport =
assignments (Source_: [TypeScript 5.8](TypeScript: Documentation - TypeScript 5.8))
Luckily the TypeScript developers introduced a new compiler option, --erasableSyntaxOnly
, which prevents the use of unsupported constructs in your code.
How to migrate code using the enum
- keyword
JavaScript lacks an enum
keyword, requiring the TypeScript compiler to transform it. Consequently, Node.js cannot remove it via type-stripping. Consider this example:
export enum OptionTypesEnum {
Option1 = "Option 1",
Option2 = "Option 2",
Option3 = "Option 3"
}
To avoid using the enum
keyword, you can rewrite the code as follows to achieve the same result:
export const OptionTypes = {
Option1: "Option 1",
Option2: "Option 2",
Option3: "Option 3"
} as const;
export type OptionTypes = keyof typeof OptionTypes;
Note: There is a TC39 proposal to introduce the keyword to the JavaScript Standard, however it is stuck in Stage 0 for the last 7 years.
Conclusion
This feature was previously introduced with Deno and Bun, but its addition to Node.js is likely to have a significant impact on future JavaScript development. With this advancement, there’s little reason to write backend JavaScript code without types.
Let’s hope we will soon see a similar feature in the browser!